ragbits docs#
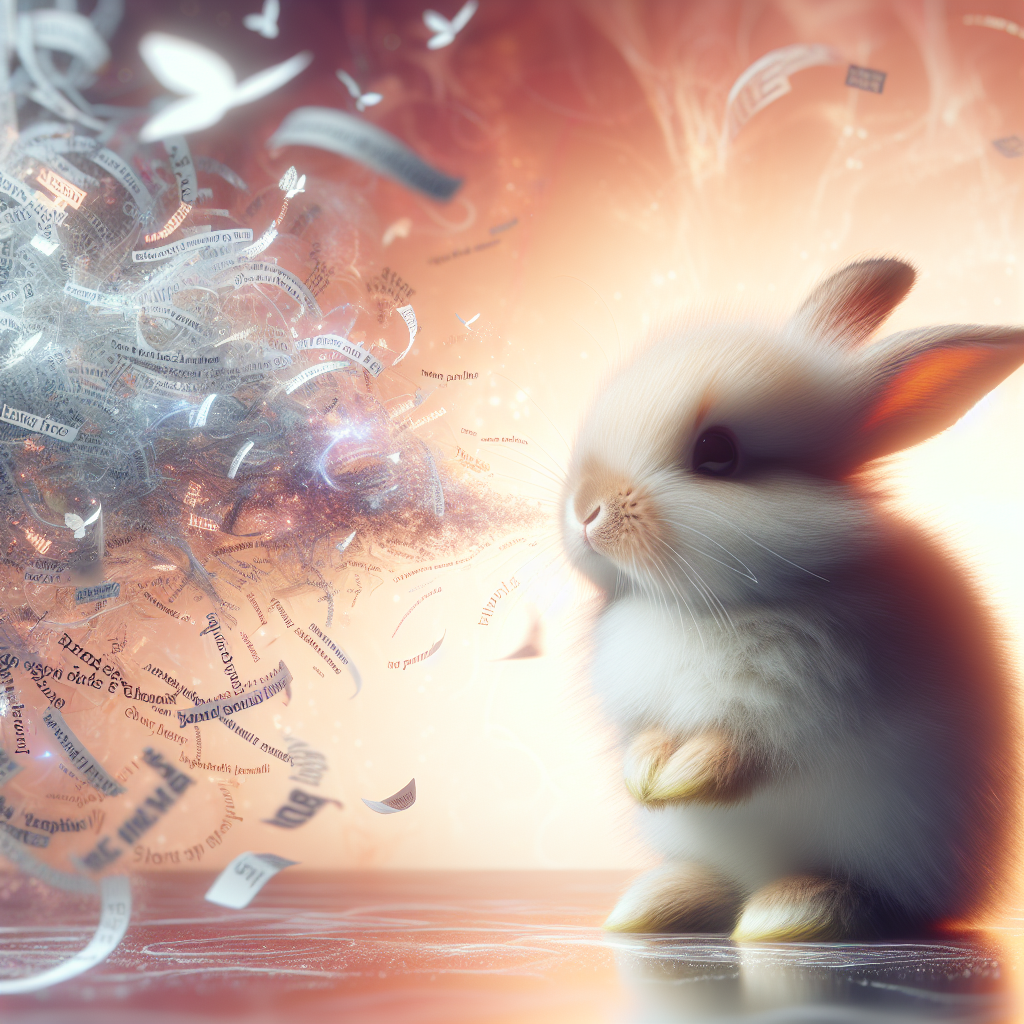
Building blocks for rapid development of GenAI applications.
ragbits is a Python package that offers essential "bits" for building powerful Retrieval-Augmented Generation (RAG) applications.
ragbits prioritizes an exceptional developer experience by providing a simple and intuitive API. It also includes a comprehensive set of tools for seamlessly building, testing, and deploying your RAG applications efficiently.
Installation#
You can install the latest version of ragbits using pip:
Additionally, you can install one of the extensions to ragbits:
ragbits[document-search]
- provides tools for building document search applications.
Quickstart#
To build the simplest documents search, you can use the following code snippet:
import asyncio
from ragbits.core.embeddings import LiteLLMEmbeddings
from ragbits.core.vector_store import InMemoryVectorStore
from ragbits.document_search import DocumentSearch
from ragbits.document_search.documents.document import DocumentMeta
documents = [
DocumentMeta.create_text_document_from_literal("RIP boiled water. You will be mist."),
DocumentMeta.create_text_document_from_literal(
"Why doesn't James Bond fart in bed? Because it would blow his cover."
),
DocumentMeta.create_text_document_from_literal(
"Why programmers don't like to swim? Because they're scared of the floating points."
),
]
async def main():
document_search = DocumentSearch(embedder=LiteLLMEmbeddings(), vector_store=InMemoryVectorStore())
await document_search.ingest(documents)
return await document_search.search("I'm boiling my water and I need a joke")
if __name__ == "__main__":
print(asyncio.run(main()))